Repeat
The repeat
concept of the QMOD language is just like a classical for loop but for quantum objects. That is, when one wants to repeat a quantum operation several times, e.g. to do a bit-wise operation, they can just use the repeat
concept of QMOD.
With the Classiq Python SDK package repeat
is accessed as a function that has 2 arguments:
count
:int
orCInt
- how many times to repeat the operation;iteration
:QCallable
- what operation to repeat;
The argmuent that is passed as the iteration
is a function by itself. Therefore it leverages the concept of lambda functions in Python (read more here).
In the following example, two quantum states \(|\psi \rangle\) and \(| \phi \rangle\) are initialized with 5 qubits each. Then an Hadamard gate is applied on all the qubits of \(|\psi \rangle\), i.e. \(H^{\otimes 5}|\psi \rangle\), and a NOT gate on all the qubits of \(|\phi \rangle\), i.e. \(X^{\otimes 5}|\phi \rangle\).
Finally, using the repeat
function, a bit-wise SWAP
is performed between \(|\phi \rangle\) and \(|\psi \rangle\).
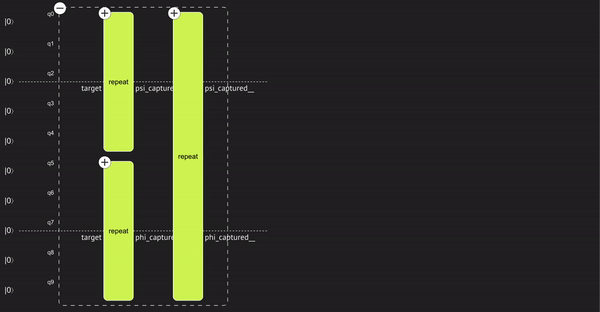
from classiq import (
SWAP,
H,
Output,
QArray,
QBit,
X,
allocate,
apply_to_all,
create_model,
qfunc,
repeat,
show,
synthesize,
)
@qfunc
def main(psi: Output[QArray[QBit]], phi: Output[QArray[QBit]]):
allocate(num_qubits=5, out=psi)
allocate(num_qubits=5, out=phi)
apply_to_all(H, psi)
apply_to_all(X, phi)
repeat(count=psi.len, iteration=lambda i: SWAP(phi[i], psi[i]))
qprog = synthesize(create_model(main))
show(qprog)
Opening: https://platform.classiq.io/circuit/5b8e48b5-93c5-4068-8ce7-3ba10b2a814b?version=0.41.0.dev39%2B79c8fd0855
The native QMOD version of the above code:
// Repeat Example
qfunc main(output psi: qbit[], output phi: qbit[]) {
allocate<5>(psi);
allocate<5>(phi);
apply_to_all<H>(psi);
apply_to_all<X>(phi);
repeat(i:psi.len) {
SWAP(phi[i], psi[i]);
}
}
from classiq import write_qmod
write_qmod(create_model(main), "repeat")