Synthesis Tutorial
Classiq's synthesis engine takes a high-level model written in the Qmod language, and compiles it into an executable gate-level circuit.
When mapping high-level functionality to concrete circuits, there may be many different but equivalent possible implementations that reflect tradeoffs in the overall depth, width, gate counts, etc. For example, implementing a multi-controlled-not operation can be shallower in gates given more auxiliary qubits. Choosing the best implementation for a specific operation instance depends on the overall constraints and objectives, as well as the specific structure of the quantum program.
Let's look at a simple model, and use Classiq's synthesis engine to compile it given different optimization objectives.
from classiq import *
@qfunc
def main(x: Output[QNum[3]], y: Output[QNum]) -> None:
allocate(x)
hadamard_transform(x)
y |= x**2 + 1
First, let's synthesize to optimize on circuit depth, i.e. to minimize the longest path formed by gates in the circuit (and hence affects the required coherence time).
qprog_opt_depth = synthesize(
model=main,
constraints=Constraints(optimization_parameter=OptimizationParameter.DEPTH)
)
We can inspect the resulting circuit using Classiq's web visualization:
show(qprog_opt_depth)
Opening: https://platform.classiq.io/circuit/2tFsFhhCaOu0oxISGTKjXSkbSty?version=0.68.0
On the left side menu, under 'Program info', you should see the resulting depth, width and gate-count:
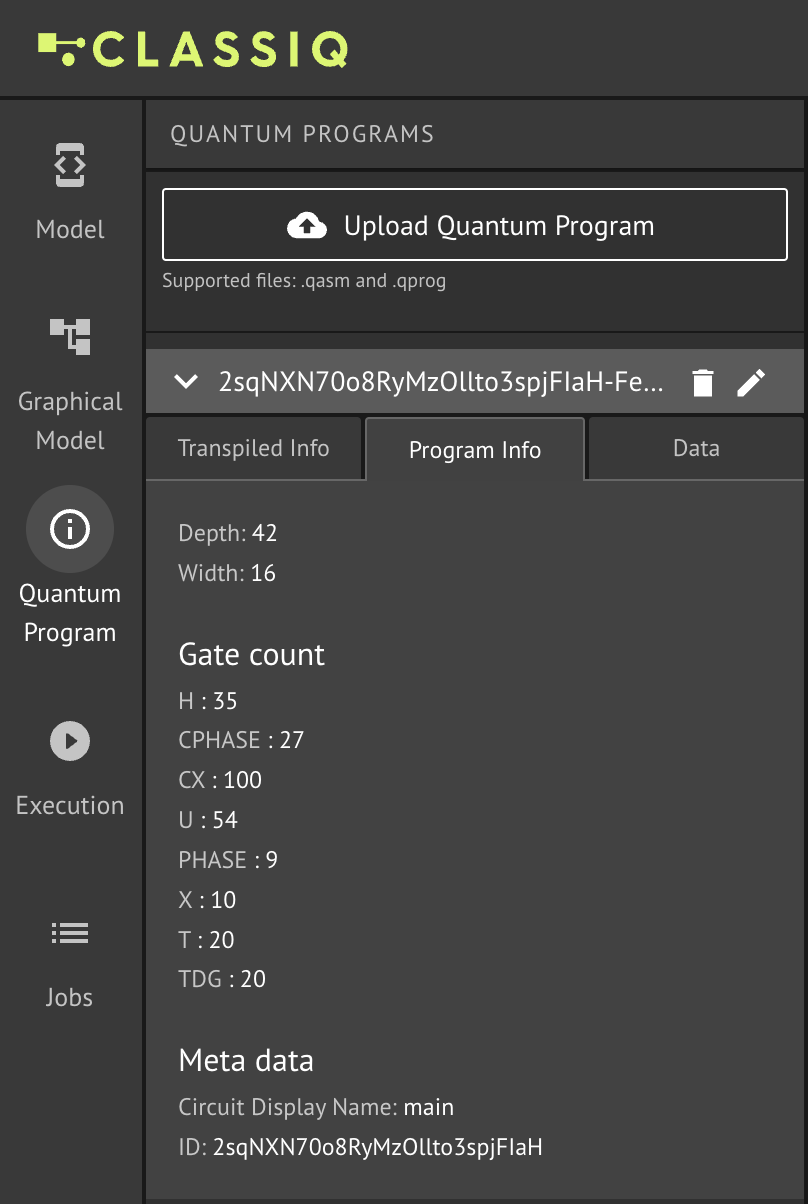
The resulting depth and width are 42 and 16, respectively.
Now, let's synthesize to optimize width, i.e to minimize the number of qubits used.
qprog_opt_width = synthesize(
model=main,
constraints=Constraints(optimization_parameter=OptimizationParameter.WIDTH)
)
Inspect the resulting circuit:
show(qprog_opt_width)
Opening: https://platform.classiq.io/circuit/2tFsGKc7EHcx0fX3oXREB18FTjd?version=0.68.0
The new depth and width are 47 and 9, respectively. As expected, we "pay" with extra depth for an implementation that uses less qubits.
Exercise
Create your own main
function and synthesize it with different constraints.
Note that OptimizationParameter
is only one kind of configuration possible. Classiq synthesis engine also supports rigid constraints of max_width
, max_depth
and max_gate_count
.